The User Interface Options (UI Options) component allows users to transform the presentation of the user interface
and content resources so that they are personalized to the individual user's needs.
UI Options does three things:
- places a preferences editor dialog with a set of panels in a collapsible panel at the top of the page, accessible
through a button in the upper right corner of the page;
- instantiates a cookie-based Settings Store for storing the user's preferences; and
- acts upon the user's preferences.
UI Options is a convenient way to add a simple separated-panel preferences editor to any page. The interface will
automatically support the set of "starter" preferences provided by the Preferences Framework,
in their default configuration.
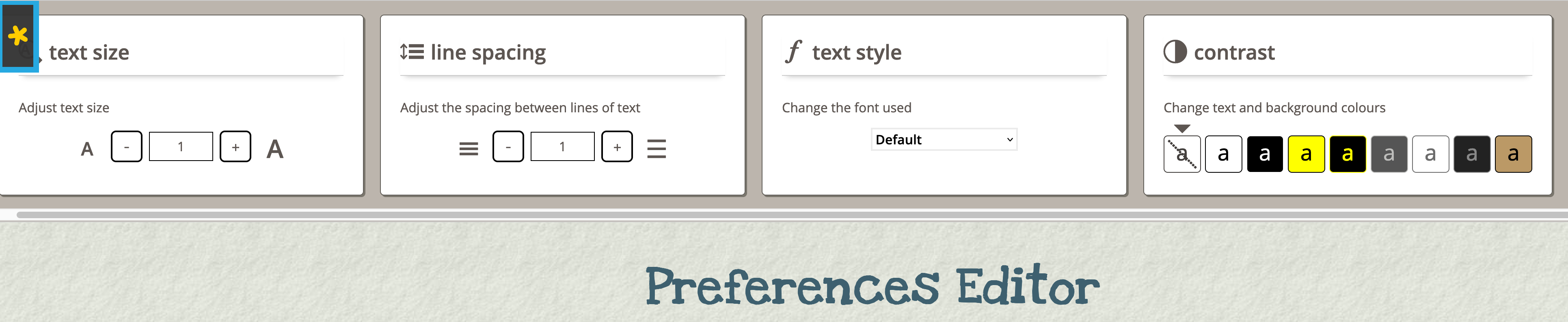
Creator
Use the following function to create a UI Options component:
Method |
fluid.uiOptions(container, options);
|
Description |
Instantiate a separated panel version of the UI Options component, which displays the controls in a
sliding panel at the top of the page.
|
Parameters |
- container
-
A CSS-based selectors, single-element jQuery object, or DOM element that identifies the root DOM
node where the UI Options interface should be placed.
- options
-
An optional data structure that configures the UI Options component, as described below.
|
Returns |
The UI Options component |
Examples |
var myUIO = fluid.uiOptions("#myContainer", {
auxiliarySchema: {
terms: {
"templatePrefix": "lib/infusion/src/framework/preferences/html"",
"messagePrefix": "lib/infusion/src/framework/preferences/messages""
},
"fluid.prefs.tableOfContents": {
enactor: {
"tocTemplate": "lib/infusion/src/components/tableOfContents/html/TableOfContents.html"",
"tocMessage": "lib/infusion/src/framework/preferences/messages/tableOfContents-enactor.json""
}
}
}
});
|
Notes |
UI Options uses the page itself as a live "preview:" As users adjust controls, the page is modified
accordingly.
|
Options
The second argument to the creator function is the options argument. This is a JavaScript object containing name/value
pairs: The name is the name of the option and the value is the desired setting. Components define their own default
values for options, but integrators can override these defaults by providing new values using the options argument. For
technical information about how options are merged with defaults, see Options Merging.
var uio = fluid.uiOptions(".myContainer", {
option1Name: option1value,
option2Name: option2value
});
Some top level options supported by UI Options are described below.
auxiliarySchema
Description |
The auxiliarySchema option allows you to configure the individual individual preferences
as well as the paths to the templates and message bundles.
|
Default |
{
"loaderGrades": ["fluid.prefs.separatedPanel"],
"generatePanelContainers": true,
"template": "%templatePrefix/SeparatedPanelPrefsEditor.html",
"message": "%messagePrefix/prefsEditor.json",
"terms": {
"templatePrefix": "../../framework/preferences/html",
"messagePrefix": "../../framework/preferences/messages"
}
}
|
Example |
fluid.uiOptions("#myContainer", {
auxiliarySchema: {
terms: {
"templatePrefix": "lib/infusion/src/framework/preferences/html"",
"messagePrefix": "lib/infusion/src/framework/preferences/messages""
},
"fluid.prefs.tableOfContents": {
enactor: {
"tocTemplate": "lib/infusion/src/components/tableOfContents/html/TableOfContents.html"",
"tocMessage": "lib/infusion/src/framework/preferences/messages/tableOfContents-enactor.json""
}
}
}
});
|
buildType
Description |
A typical instance of UI Options includes the preference editor, enhancer, and store. The
buildType option allows you to instantiate a subset. For example, if you will be
implementing the user interface independently. Due to dependencies, each option
(store , enhancer , prefsEditor ) will include its predecessors.
|
Default |
"prefsEditor"
|
Example |
fluid.uiOptions("#myContainer", {
buildType: "enhancer"
});
|
enhancer
Description |
The enhancer option allows you to specify a data structure to config the
enhancer component.
|
Default |
null |
Example |
fluid.uiOptions.prefsEditor("#myContainer", {
enhancer: {
invokers: {
updateModel: "my.update.function"
}
}
});
|
enhancerType
Description |
The enhancerType option allows you to specify a custom enhancer grade component.
|
Default |
"fluid.pageEnhancer"
|
Example |
fluid.uiOptions("#myContainer", {
enhancerType: "my.enhancer"
});
|
preferences
Description |
The preferences takes an array of preference names, indicating the preferences to include.
|
Default |
[
"fluid.prefs.textSize",
"fluid.prefs.lineSpace",
"fluid.prefs.textFont",
"fluid.prefs.contrast",
"fluid.prefs.tableOfContents",
"fluid.prefs.enhanceInputs"
]
|
Example |
fluid.uiOptions.prefsEditor("#myContainer", {
preferences: [
"fluid.prefs.letterSpace",
"fluid.prefs.wordSpace",
]
});
|
prefsEditor
Description |
The prefsEditor option allows you to specify a data structure to config the
prefsEditor component.
|
Default |
null |
Example |
fluid.uiOptions.prefsEditor("#myContainer", {
prefsEditor: {
listeners: {
onReady: function (internalEditor) {...}
onReset: function (internalEditor) {...}
}
}
});
|
prefsEditorLoader
Description |
The prefsEditorLoader option allows you to specify a data structure to config the
prefsEditorLoader component.
|
Default |
null |
Example |
fluid.uiOptions.prefsEditor("#myContainer", {
prefsEditorLoader: {
lazyLoad: true
}
});
|
storeType
Description |
The storeType option allows you to specify a custom store grade component.
|
Default |
"fluid.prefs.globalSettingsStore" |
Example |
fluid.uiOptions.prefsEditor("#myContainer", {
storeType: "myNamespace.mySettingsStore"
});
|
See also |
Cookie Settings Store
|
Supported Events
Listeners can be attached to any supported events through a component's listeners
option. Values can be a function
reference (not a string function name) or an anonymous function definition, as illustrated below:
var myComponent = component.name("#myContainerID", {
listeners: {
eventName1: functionName,
eventName2: function (params) {
}
}
});
For information on the different types of events, see Infusion Event System.
onReady
Description |
This event fires when the UI Options component is fully instantiated, rendered and ready to use.
|
Type |
default |
Parameters |
- uio
- The instantiated UI Options component.
|
onPrefsEditorReady
Description |
This event fires when the UI Options interface has been rendered.
Note: use onReady if the listener needs UI Options to be both rendered
and ready to use.
|
Type |
default |
Parameters |
- prefsEditorLoader
-
The instantiated preference editor loader component.
|